Learn with
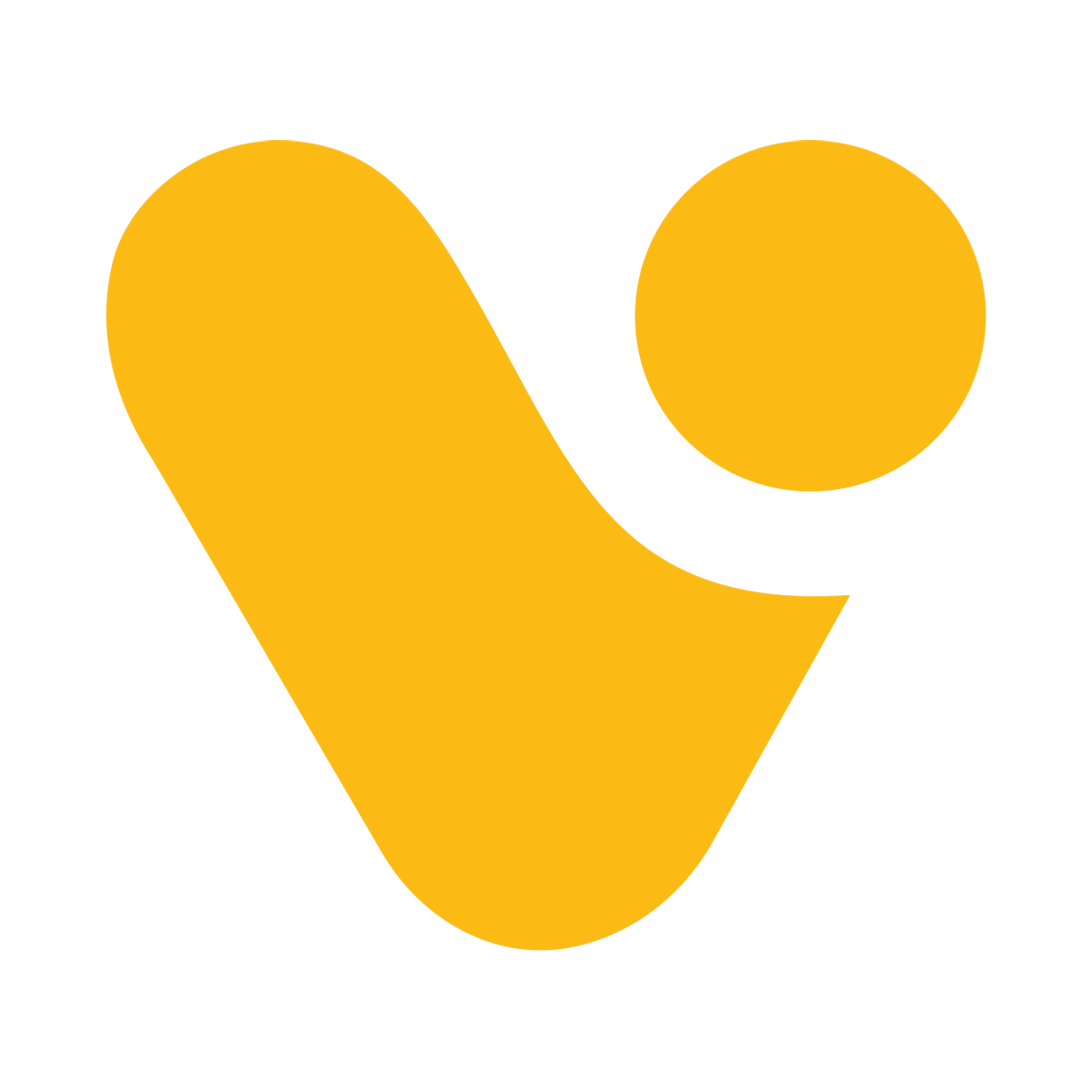
Practical Uses of Variables in Power Apps
Here are some common scenarios where variables are used effectively:
i. Navigating Between Screens
You can use a global variable to keep track of which screen the user is currently on, allowing you to control navigation.
Example:
Set(gblCurrentScreen, "HomeScreen"); Navigate(scrHomeScreen);
ii. Storing User Inputs
Context variables are great for storing the value of a form field or other user input temporarily, which you can then use to patch to a data source or process further.
Example:
UpdateContext({locUserInput: txtUserInput.Text});
iii. Handling Conditional Visibility
Variables can control the visibility of elements on the screen based on user actions. For example, you can hide or show elements based on whether a checkbox is selected.
Example:
UpdateContext({locShowPanel: tglShowPanel.Value});
Set the visibility property of the panel to:
locShowPanel
iv. Storing and Displaying Lists
Collections allow you to temporarily store and manipulate lists of data without needing to update your data source constantly. For instance, you can use collections to store a list of items that will later be saved to a database.
Example:
Collect(colShoppingCart, {ProductID: 1, ProductName: "Laptop", Quantity: 1});
v. User Session Data
You might use a global variable to store the logged-in user’s role or other details so that you can display personalized information or control which features are accessible.
Example:
Set(gblCurrentUser, User());
3. Best Practices for Using Variables
- Limit Global Variables: Avoid using too many global variables, as they can make your app more difficult to debug and maintain. Use context variables for screen-specific data.
- Use Collections for Tables: If you're working with lists or tables, collections are a better choice than trying to store lists in global or context variables.
- Scope Awareness: Be mindful of the scope of your variables. Use global variables (gbl) when the data needs to persist across screens, and use context variables (loc) when data is specific to a single screen.
By following these Power Apps coding standards and practices, your app will be more maintainable, readable, and efficient.
Here’s how we’re applying various Power Apps features—global variables, context variables, and collections—in our application with specific examples:
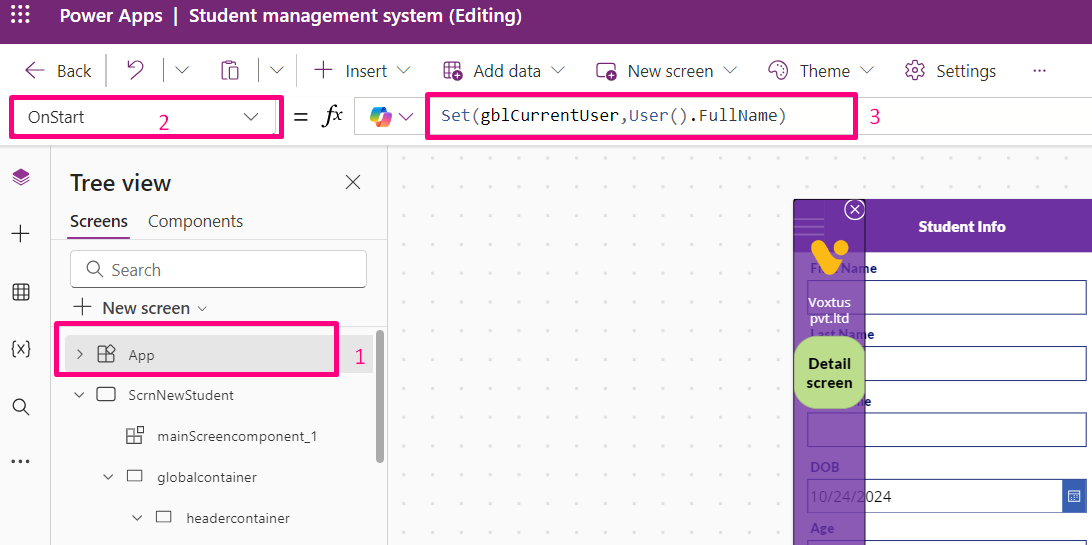
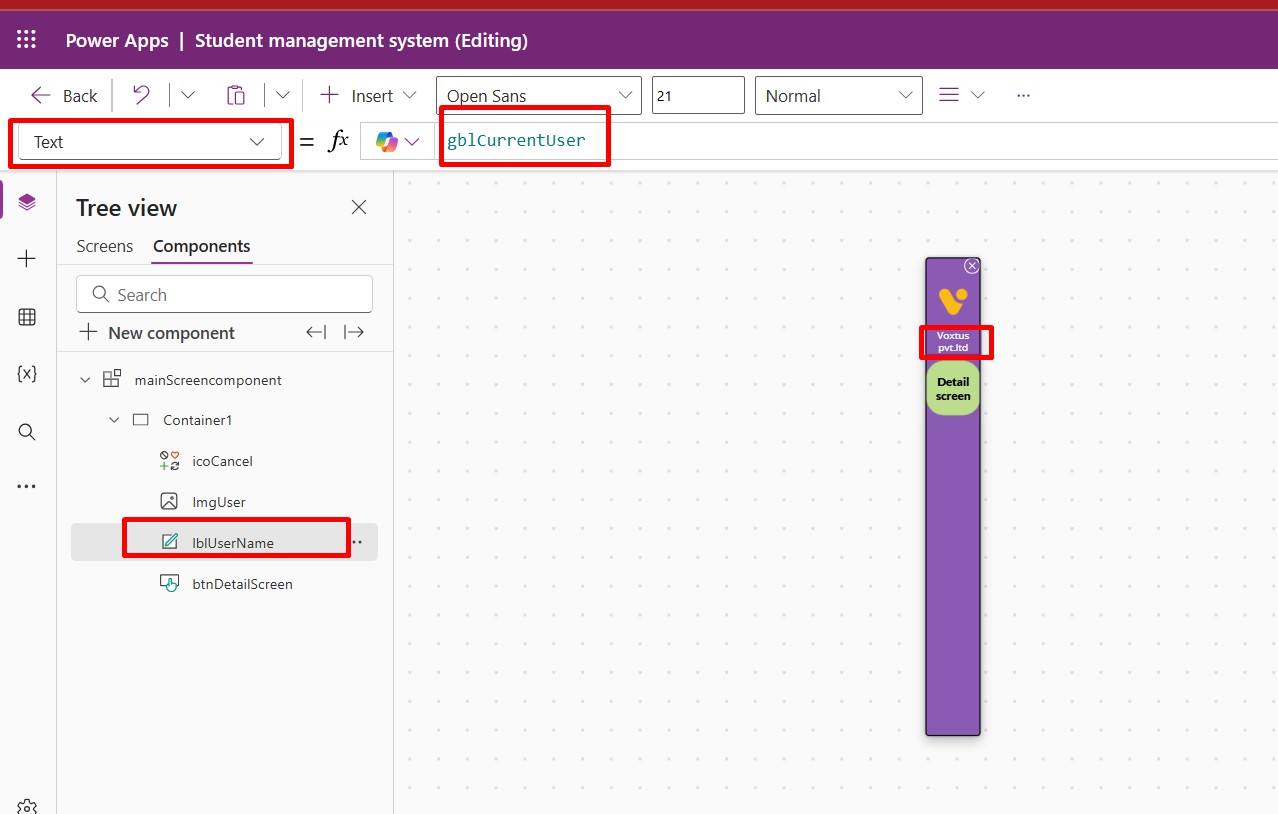
1. Using a Global Variable
To manage data accessible throughout the app, we initialize a global variable in the App OnStart property. Here’s an example:
Set(gblCurrentUser, User().FullName)
This variable, gblCurrentUser, stores the full name of the currently logged-in user. We then use this variable on the app’s home screen, specifically in the left navigation panel, to display the user’s name.
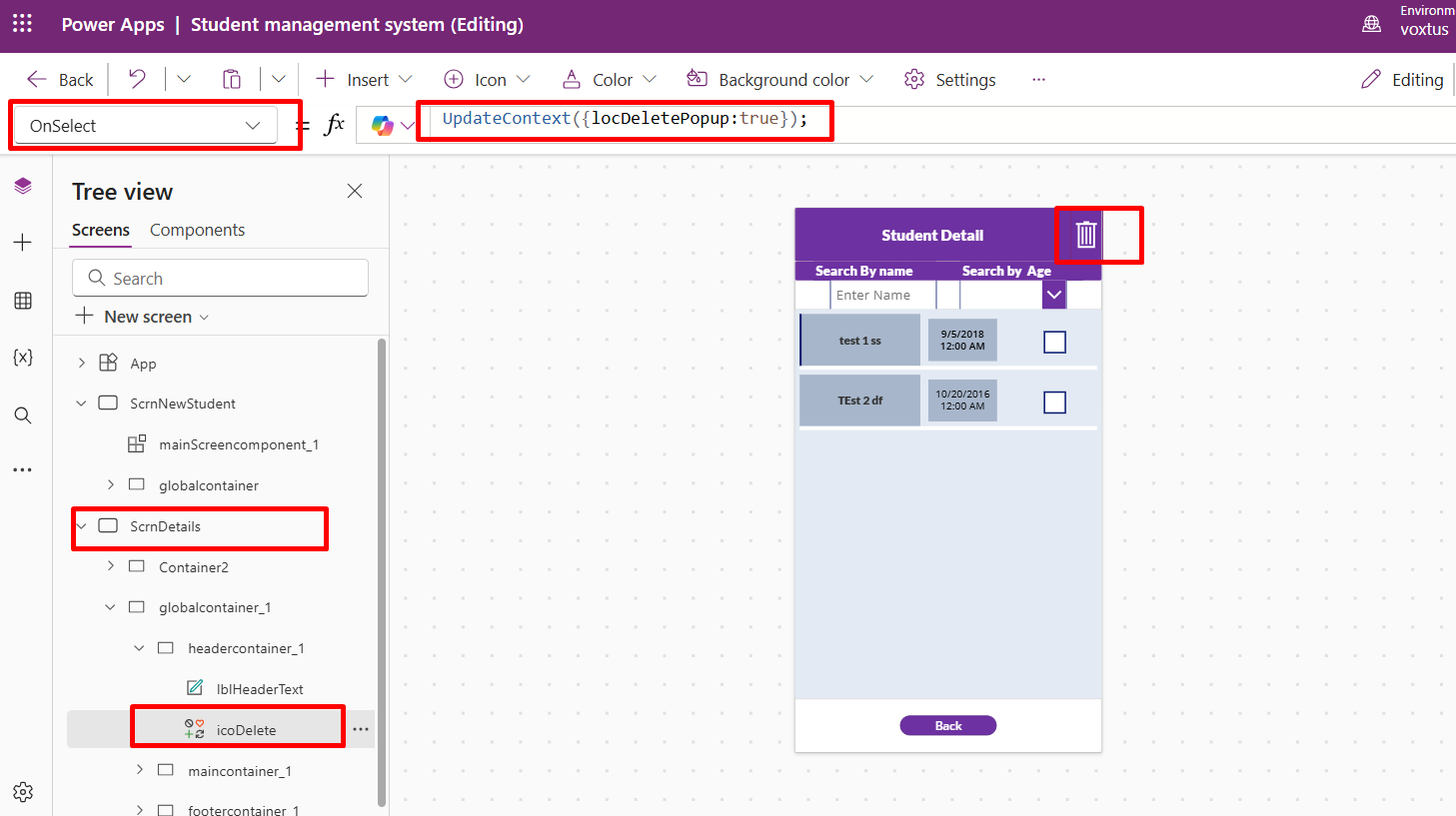
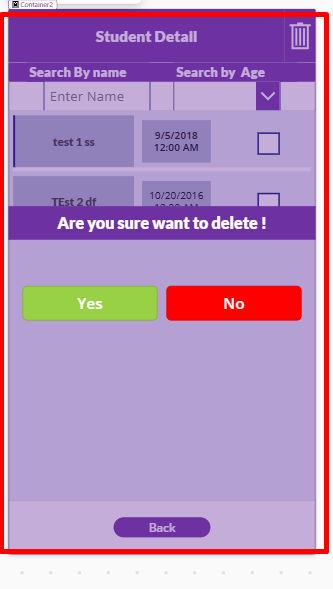
2. Using a Context Variable for Pop-Up Screens
Context variables are local to each screen, making them ideal for managing pop-ups or temporary elements. Here’s how we use a context variable for a delete confirmation popup:
-
Step 1: On the detail screen, the user clicks the delete icon, where the OnSelect property is set as follows:
UpdateContext({locDeletePopup: true})
This action sets locDeletePopup to true, triggering the visibility of the popup screen.
- Step 2: On the popup screen, the Visible property is set to check locDeletePopup. When locDeletePopup is true, the popup becomes visible. The user can then proceed with the deletion or cancel the operation.
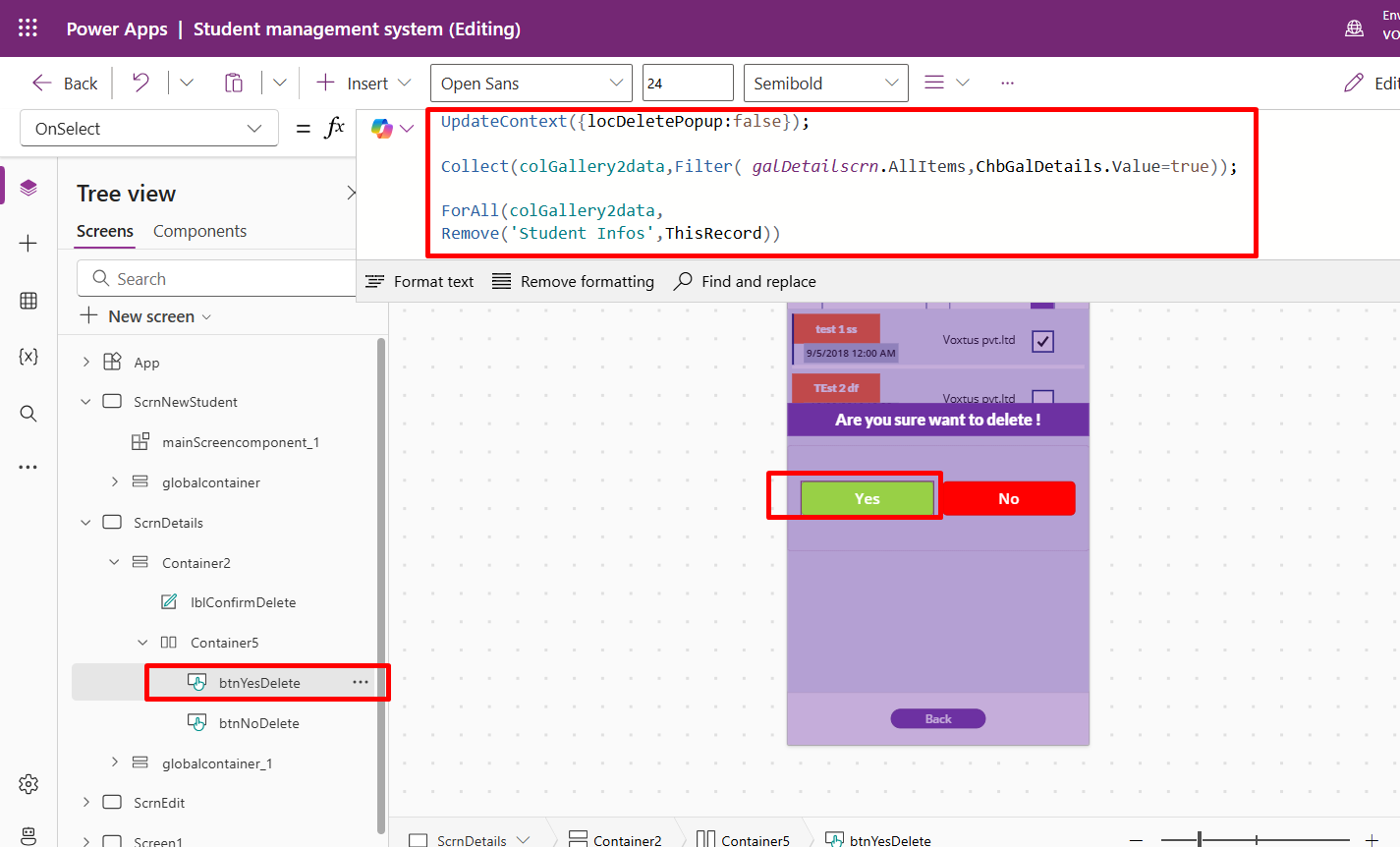
3. Using a Collection to Handle Multiple Selections
Collections are used to manage and manipulate data locally in the app. Here’s how we use a collection for batch deletion:
- Step 1: On the detail screen, we add a checkbox in each item within the gallery, allowing the user to select multiple items for deletion.
-
Step 2: The delete icon triggers a popup screen asking the user to confirm the deletion. If the user clicks "Yes," the app executes the following code:
UpdateContext({locDeletePopup: false}); Collect(colGallery2data, Filter(galDetailscrn.AllItems, ChbGalDetails.Value = true)); ForAll(colGallery2data, Remove('Student Infos', ThisRecord));
-
Explanation:
- UpdateContext({locDeletePopup: false}) closes the popup.
- Collect(colGallery2data, Filter(Gallery2.AllItems, Checkbox1.Value = true)) creates a collection (colGallery2data) that stores all gallery items where the checkbox is selected (Checkbox1.Value = true).
- ForAll(colGallery2data, Remove('Student Infos', ThisRecord)) iterates over colGallery2data and removes each selected record from the 'Student Infos' data source.
-
Explanation: